--Originally published at |Blogging through my thoughts|
**Updated post**
Hello friends !
For this task , my classmates and I were instructed to create a program that will prompt the user for a temperature in Fahrenheit and them convert it to Celsius. The formula that made the conversion possible was C = 5 ∗ (F − 32)/9 (where F = Fahrenheit).
The resources that helped me were:
-cpluplus.com
-How to think like a computer scientist.
I added some extra steps to show more information to the user , depending on the number (x) given , like if:
-Water boils at x temperature.
-Water is in its solid state, ice.
-Water, in its solid state, becomes liquid due to its melting point.
-Water does not boil at this temperature.
It was a fun and entertaining task.

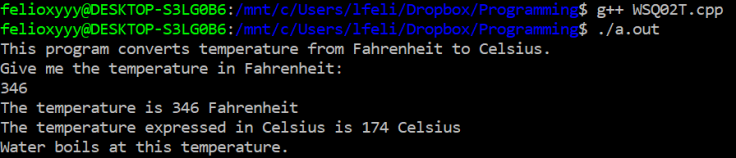
