--Originally published at Programming
First of all, you could learn more about Fibonacci in Wikipedia.
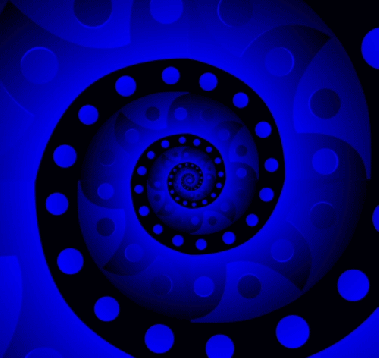
With a function we will return the Fibonacci number. For example, if we introduce 12, it returns 144, because in the sequence this is the value of the position.
But in this sequence, the numbers before 2 are the same. Remember that and we can start making our code. Let’s see:
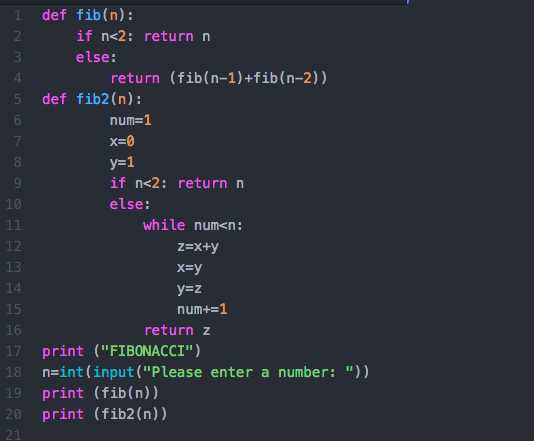
Well, look that we implemented two solutions. The first one is with recursion, we defined a function, then an if to consider what about if the user choose a number before 2. If not, we return the result of fibonacci’s formula.
The second one, is the same, but we are using a loop. For this we declared our counter like num, it starts at 1 because the serie starts at this number. After that declare x like zero because it doesn´t count for the first sum we want to do, and y like one, because the serie starts here. If the number is before the 2, you should return the same number, but if not we start with the loop until while the counter is smaller than input. Inside here, z will be the first value of the serie plus y, the second one. Then, they will change as you see in the code to continue moving inside the line of numbers. Finally here we return just z, that works like the final result.
Just print, as you see both ways give us the same, so they will give you exactly the same every time, but we are printing both to confirm.
This is how it runs:
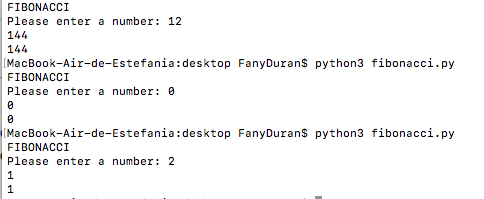
Hope it helps 