--Originally published at Programming
Hello! Today we are doing a factorial calculator. This is about calculate the factorial of a number, asking the user for a non-negative integer. If you don’t know is the factorial or you want to refresh, you can know about it in Wikipedia-Factorial.
Well, as usual, here is the code: (and then I will explain)
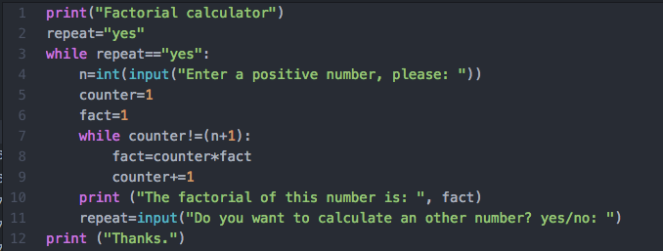
First we print an introduction phrase for the user. The objetive of this exercise is to use loops. To start the loop our coindition needs to be true, thats why we declared repeat as “yes” and the we start the loop.
Inside this loop, we ask for the number like an int. Then we inicialize the counter like one and the fact too, fact is the number that will be multipliying and incrementing. For that happens we made another loop. The condition as we see is that the counter is different than the number (that the user give us) plus one. This way then we see that fact will be multiplicating the counter and after that it will increase one.
After this math operations we print the result with a sentence to advise, and finally we ask the user if he want to repeat the cycle.
If yes, all start again. If no, we just print Thanks.
And that’s it. This is how it runs:

Hope it helps 