--Originally published at S' Nami Bog. Servitas Vitae
Follow this short and quick tutorial on how to use WASM!
From top to bottom- we have 10 different steps in order to get a Hello World from C without writing anything in our js file.
- We will select an Empty C Project although you can use C++ and Rust for using WASM aka High Level Languages.
- You will get three files, main.c main.html and main.js
- If you open the js file- you will see that the frist line of code is fetching the WASM that will later be instantiated into the wasm code
- As you can see we cleaned up the code and started using async
- Then we will run the code and get the result for output build running and build is complete
- After running it for the ou you will see a main.wasm file
- By checking the console you will see a related object named instance and module
- In the c file we have a reference to the js that calls the function
- We will next create a simple c code to run the Hello World
- Sadly we will have a null pointer so we will fix that
- Next step will be to get the memory buffer
- Fnally we will create a for function in order to get our string hello world variable
- Calling it and getting ID will print our Hello World named WASM
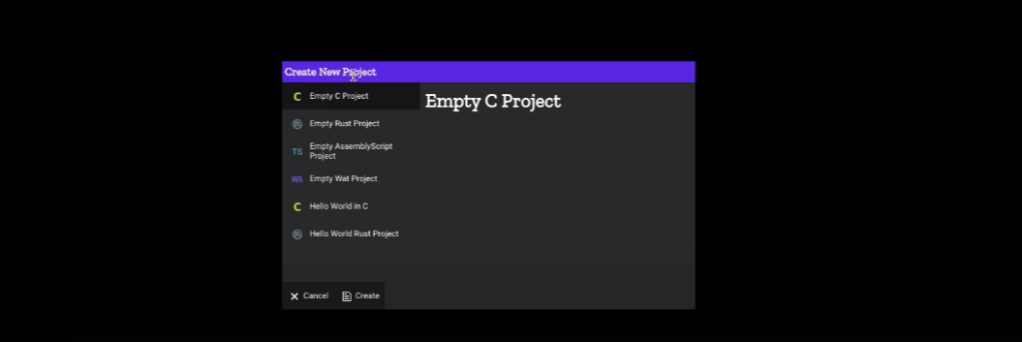


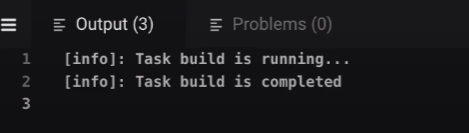
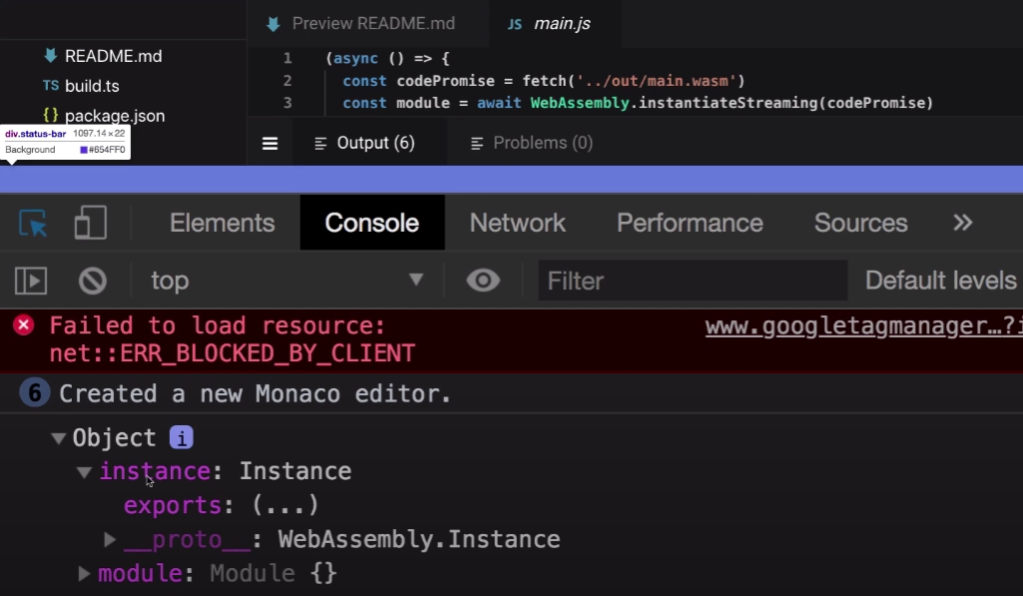
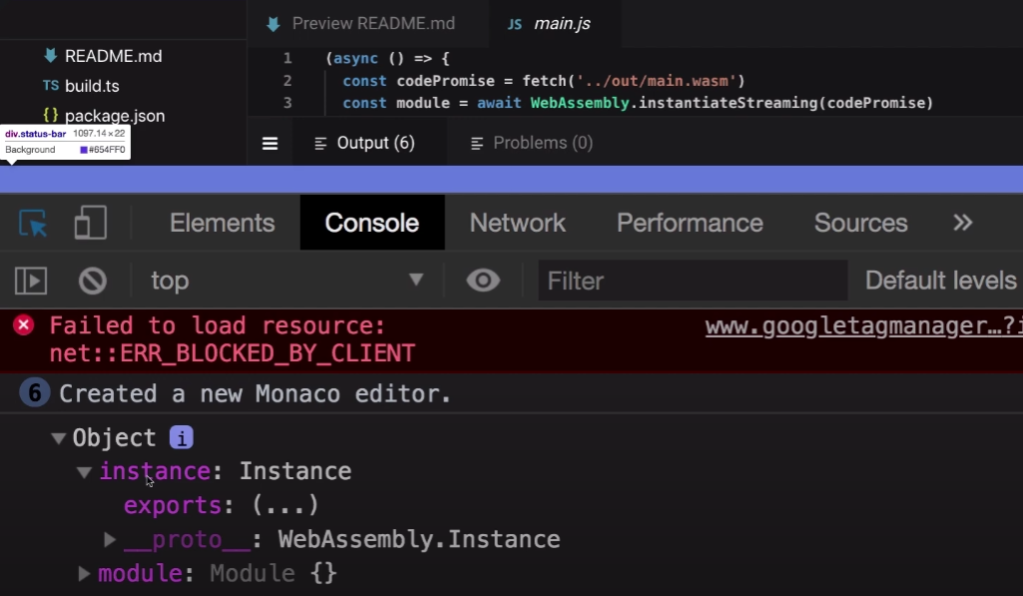
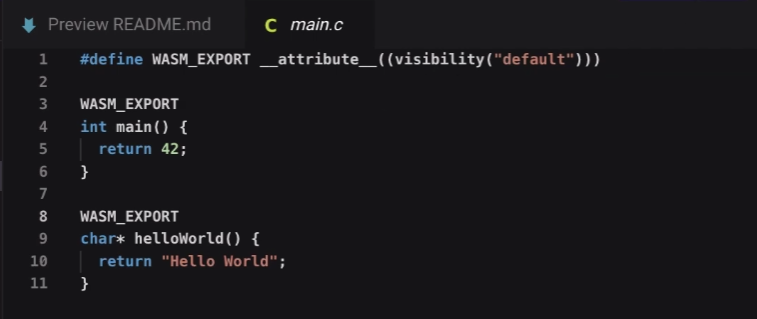

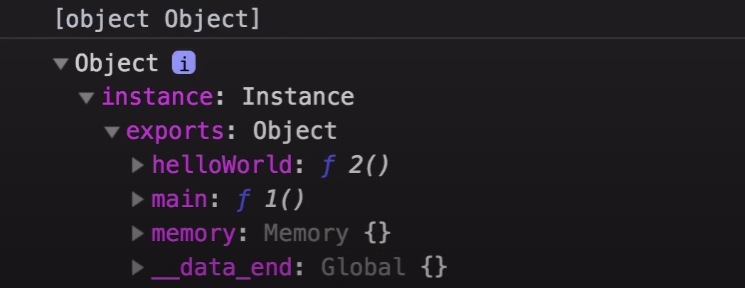
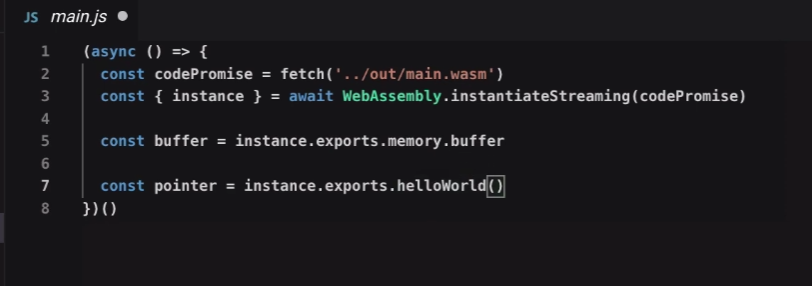
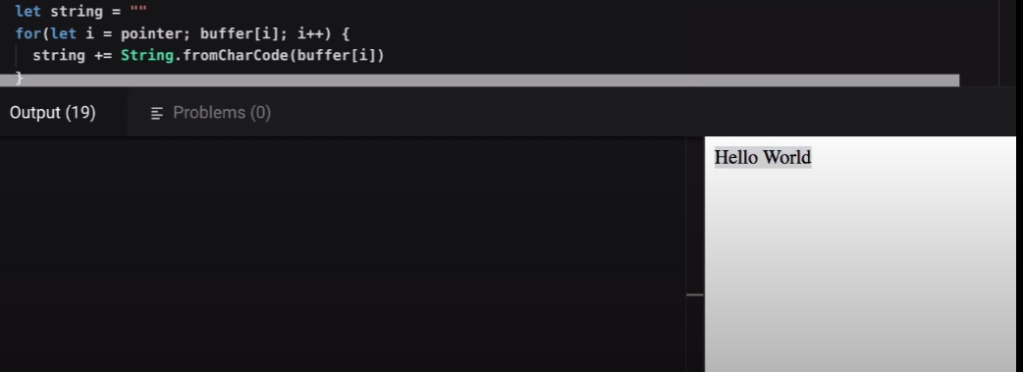