--Originally published at GTO
This is the first program I made after setting up my software and programed “Hello World” and since that I have been reading and searching information about programming in C++ because I have never used it before, I do have a little bit of experience programming but not in C++, it has not been very difficult neither easy.
This program is very basic, teacher Ken asked us to made something that: Ask the user for two integer values, then use those two values to calculate and show the following:
- The sum of the two numbers.
- The difference of the two numbers.
- The product of the two numbers.
- The integer based division of the two numbers (so no decimal point). First divided by second.
- The remainder of integer division of the two numbers.
So this is what I made:
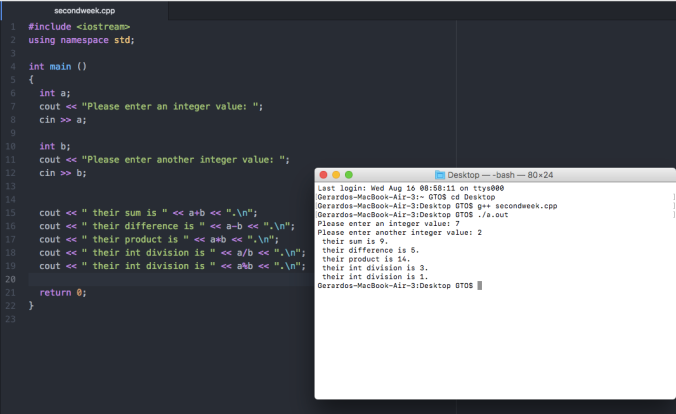
I already had an idea of how to start something in C++ thanks to “Hello World” but I needed to look up for information about the variables, the operators and how to show the results in the book: “How to Think Like a Computer Scientist, C++ Version”, Downey, Allen B. 2012.
My code is:
#include <iostream>
using namespace std;
int main ()
{
int a;
cout << “Please enter an integer value: “;
cin >> a;
int b;
cout << “Please enter another integer value: “;
cin >> b;
cout << ” their sum is ” << a+b << “.\n”; //The sum of the two numbers.
cout << ” their difference is ” << a-b << “.\n”; //The difference of the two numbers.
cout << ” their product is ” << a*b << “.\n”; //The product of the two numbers.
cout << ” their int division is ” << a/b << “.\n”; //The integer based division of the
Continue reading "A little bit of math with programming" →