JUnit is an unit testing framework for the Java programming language.
From tutorialspints:
JUnit promotes the idea of “first testing then coding”, which emphasizes on setting up the test data for a piece of code that can be tested first and then implemented
This approach is kind of test a little and code a little because you don’t test the whole project but instead part of the software such as individual method to ensure that is working properly. It also increase the productivity of the programmer and reduces the stress; this because you do not leave any problems or things like “fix it later” in the program.
In java, JUnit is used as a library by importing the necessary classes an then creating a constructor of the test and implementing methods that call an instance of the class you want to test that are used to execute specific method of the tested class. The goal for each of those testing methods is to assert the expect behavior comparing the the expected value and the actual value. You can use any data type: from data primitive (such as int, char, float, etc.) to arrays of any type, any objects and nulls.
Many advantages of JUnit are that it doesn’t require human judgment to interpret where the failure happens, what you actually have to do is create a testing class from your class that you want to test, make some adjustments and execute it and wait until the output tells you where the error is. And if your are using an Integrated Development Environment (IDE) is more easier to do it, which is actually another advantage because JUnits is included in most of IDE such as Eclipse and NetBeans.
I will write a quick guide of how to use it
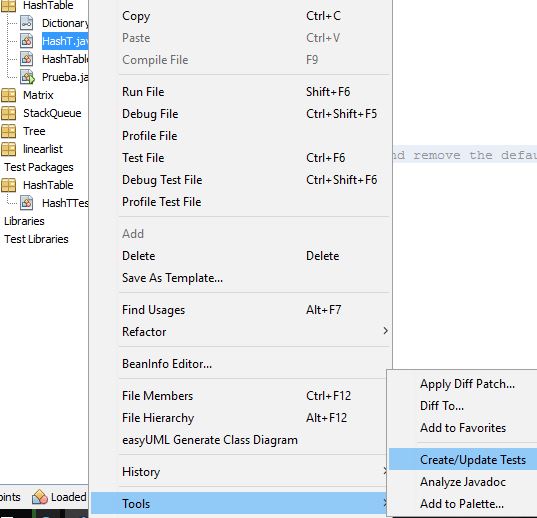
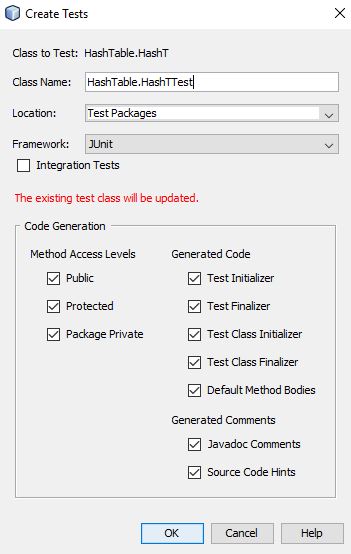
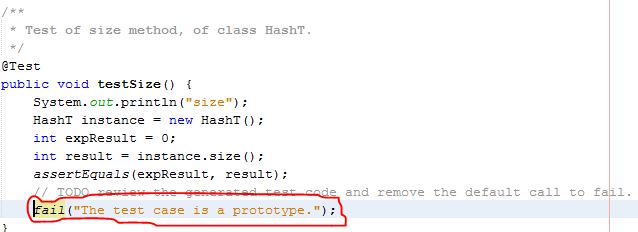
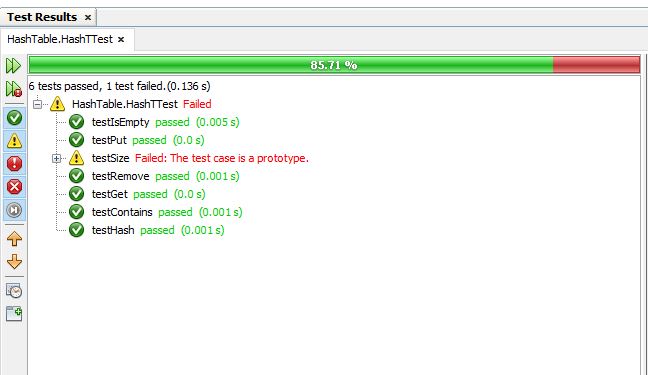
