--Originally published at Elu's Blog
For this assignment this were the instructions:
Write a program that will prompt the user for a temperature in Fahrenheit and then convert it to Celsius. You may recall that the formula is C = 5 ∗ (F − 32)/9. Modify the program to state whether or not water would boil at the temperature given.
And this is what I achieved:
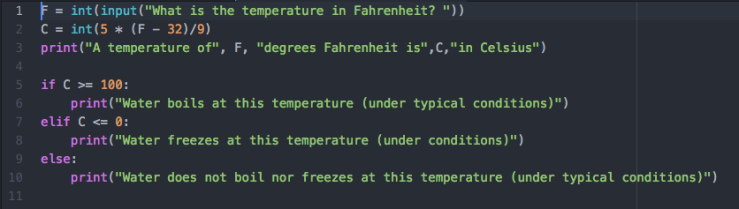
As it can be observed, there are the int() and input() functions that we already know, but I haven’t covered the if, else and elif statements. This are very easy to understand and code. When you say, “if I study, I’ll do well on my exam”, you have the conditional “if I study,” and the result “I’ll do well on my exam”. When coding, it’s really the same thing, in line 5 we can observe the conditional “if C >= 100:” (which can be read, “if C is equal or greater than 100”) and in line 6 we can observe the result ‘print(“Water boils at this temperature (under typical conditions)”)’. The “else” statement is what happens when the “if” conditional is not achieved. The “elif” works in a way that when the first conditional is not achieved, then you can have another conditional.
Also, to nest a conditional statement means to have the whole “if, elif (optional) and else” inside an “if”, an “elif” or an “else”. When you nest it it means that you write it as the “result”.
At the end, this code runs like this:

If my explanation wasn’t clear enough check this two links:
Python Course’s explanation
http://www.python-course.eu/python3_conditional_statements.php
The Basics – Python 3: Conditional Statements Example (RTE)
https://youtu.be/enEwTN0zeNk