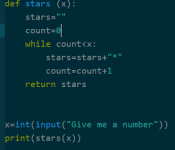
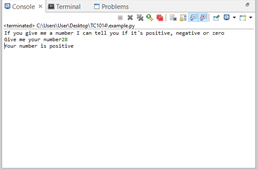
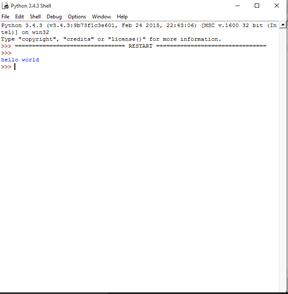
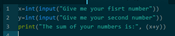
But variables are not just text, they can also be numbers. These numbers can be float numbers or integers. Float numbers are the ones that have decimal values, and integers are the ones without decimal values.
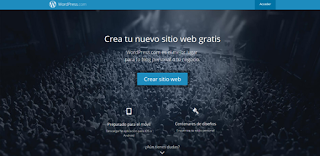
Your first step is to click on the following link: www.blogger.com
This program asks the user for a range of number and gives the sum of each one of the numbers contained in the range.
This program selects a random number between 1 and 100 and the used need to guess it. Once they guess it, the program will display the number of attempts the user had.