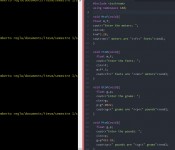
Here a program used for convert some units, using switch case an functions: The main code: https://github.com/hrglez/TC1017/blob/master/Units Thank you. Have a good day.
Here a program used for convert some units, using switch case an functions: The main code: https://github.com/hrglez/TC1017/blob/master/Units Thank you. Have a good day.
Here a program used for convert some units, using switch case an functions: The main code: https://github.com/hrglez/TC1017/blob/master/Units Thank you. Have a good day.
A switch structure is used for determining a case in base of a expression. Its structure is:
switch (expression) {
case statement :
Instructions;
break;
default:
Instructions;
break;
This works by using the expression given to determine the case that should be executed. For instance if the expression is 1, the set of instructions that will be executed are the ones found in case 1. In case of having different expressions intended to trigger the same set of instructions it could be done by typing them in series:
case 1: case 2: …. case n:
Instructions;
break;
The break statement is important since it stops the flow of the switch and avoids that other set of instructions are executed. Another characteristic is the default statement, that is the one executed if the expression given is not found in the cases.
Here’s an image of the switch case in use and its outputs:
Thanks for watching, hope this allowed you to understand this concept.
Hey today we are going to learn how to use “elif” with a conditional : #Mastery17
Hey today we are going to learn how to use “elif” with a conditional : #Mastery17
Use of “elif” with a conditional Nesting of conditional statements
Use of “elif” with a conditional Nesting of conditional statements
The elif is a conditional used to expand options of a normal conditional of If-Else. It´s very useful when you want to check different conditions at once but at the same time you want to save time at writing the code. Lets see an example, first a program without elif:
x = input(“¿What do you want to do next?”)
if x == “exit”:
#close the program
else:
if x == “pay”:
#go to the pay window
else:
if x == “back”:
#move to the previous window
else:
if x == “next”:
#move to the next window
else:
if x == “add”:
#add the item to the shopping list
else:
print (“please write exit, pay, back, next or add”)
This program is a cheap and lazy representation of an online store. It receives an input from the user and do whatever the user writes, to do this we need to check several conditions at the same time (aka a nesting of conditions): if the user wants to exit, pay, go back, go next or add the item to the list. As you can see it took me a lot of space to write those conditions in the regular way. If the first condition didn´t work it will pass to the else that is another condition and if that condition is false it will pass the the next else that is another condition and it keeps going on until one condition is true or it reaches the final else. The easy and clean way to do it is with the elif, let´s see an example of the same program but this time with elifs:
x = input(“¿What do you want to do next?”)
if x == “exit”:
#close the program
elif x == “pay”:
#go to the pay window
elif x == “back”:
#move to the previous window
elif x == “next”:
#move to the next window
elif x == “add”:
#add the item to the shopping list
else:
print (“please write exit, pay, back, next or add”)
This program do exaclty the same but it took me less time to write it and is easier to understand than the other one. The elif is like the combination of else-if, Python reads it exactly in the same way.
Notice that each elif is used as an if, with the condition by the side, the “:” at the end and the instructions are indented. To finish the nesting of conditions we put an else just as if it were a normal if/else. If the user writes “back” the first and second conditions will be false and it will execute the code inside the second elif. Have in mind that after the program ends executing the code inside the elif it will jump to the end of the nesting and continue going, what i mean is that the program will not continue checking if the other conditions are true because one has already been executed. Also, the program reads from top to botton the conditions, not at the same time; so if two conditions are true it will only execute the first one. Let´s see an example of this:
x = 3
if x > 0:
print (“X is greater than 0”)
elif x < 10:
print (“X is less than ten”)
elif x < 0:
print (“X is a negative number”)
else:
print (“X is greater than 10”)
X is greater than cero
Well, what´s wrong with this program? What´s wrong is that no matter what number you put, it will print “X is greater than 0” or “X is a negative number” completely ignoring the other two conditions. Lets see, if we write a 9 it should print “X is less than then” but also the first condition is true so it should also print “X is greater than 0” but as a nesting of conditions can only take one condition it will go for the first one to be true si it will print “X is greater than 0”. If we write number 20 the first condition to be true will be x > 0 so it will print “X is greater than 0” again. Just in case that you write a negative number it will print “X is a negative number” but for all the other numbers the first condition will always be true first. If you want to make a good condition nesting with elif (also with else/if) always have in mind which condition you give the preference. Nothing happens if two or more conditions are true, the program can still work as you want if you put them in the right order. I recommend you to order the condition from specific to general, in that way if the specific condition is true but also the general is true the program is going to take the specific condition first. Here is the same program but this time taking all the posibilities from the specific to the general:
x =0
if x == 0:
print (“X is equal to 0”)
elif x < 10:
print (“X is less than ten”)
elif x > 10:
print (“X is greater than 10”)
else:
print (“X is a negative number or is a string”)
X is equal to 0
SWITCH es una condicional, pero más compleja ya que incluye casos para validar la expresión condicional. Si no es uno de los casos mencionados, es lo que resta. The blog that helped me (where did I get the definitions and… Continue Reading →