Hello everyone! Quiz 5 has 2 really fun exercises to develop, here is the description:
- Create a function called is_palindrome which receives a string as a parameter and returns true if that string is a palindrome, false otherwise. Remember that a palindrome is a word that is the same forward or backward. For full points your function must ignore case and must work with any character (not just letters). So (“Dad$dad” is a palindrome even though the D is capital and d is lower case).
- Create a function called find_threes that receives as a parameter a list of numbers and returns the sum of all numbers in that list that are evenly divisible by 3. So if the list was [0,4,2,6,9,8,3,12], the function would return 30 (0+6+9+3+12)
Exercise 1
Here’s the first code. Don’t freak out, I’ll explain each line.
First, we define the function. Remember that capital letters shouldn’t be considered, so we convert all the letters to lowercase. I got the advice of how to do this here.
Then, in line 4, we reverse the string. stackoverflow might help you out with this step.
Then an ‘if’ and an ‘else’ clause are inserted, because we need to compare if the string is equal to the reversed one, and determine if it’s a palindrome or not.
The rest is a piece of cake. We need an input (line 10) to ask the user for the string and we print the function.
Now let’s try the code.
GIVE ME 5, it worked.
Exercise 2
This code is really easy. Let’s develop it fast and efficiently.
I’m creating a list so I can save every number divisible by 3 in that list. This list will be used in line 12, where our function called ‘find_threes’ will do it’s work.
In line 2, we have
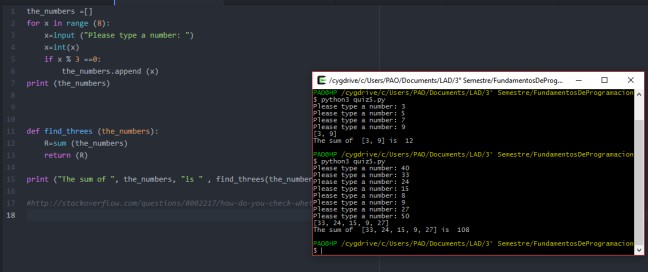


‘for’ loop that will make the selection of 8 numbers given by the user. As you can notice, each number is evaluated with the % sign with an ‘if’ clause, that decides if a number is divisible by 3 or not. I found how to do this in stackoverflow.
In line 6 we notice that if the number is a multiple of 3, it’ll be added to ‘the_numbers’.
Let’s check if this works.
YUP, it worked.
Here are my codes if you want to check them out. Have a nice week!
Quiz 5 by paogarcia2401 is licensed under a Creative Commons Attribution-ShareAlike 4.0 International License.