#TC1017 #QUIZ5
So this was hard. This, I must say, is one of my least effective codes written so far, it´s too long, buuuuuuut it works, so I´ll take it. Basically the user inputs the word, letter by letter (ineffective!) and then my code displays it the normal way and then reversed, just so the user sees it. Then it compares index by index to check if its the same.
The function .pushback was helpful for creating vectors of unknown size.
The real hard part was making it case unsensitive, oh man that took a while. Ken helped me out with a magical function and everything ran just fine. Also, mek sure to #include all necessary modules.
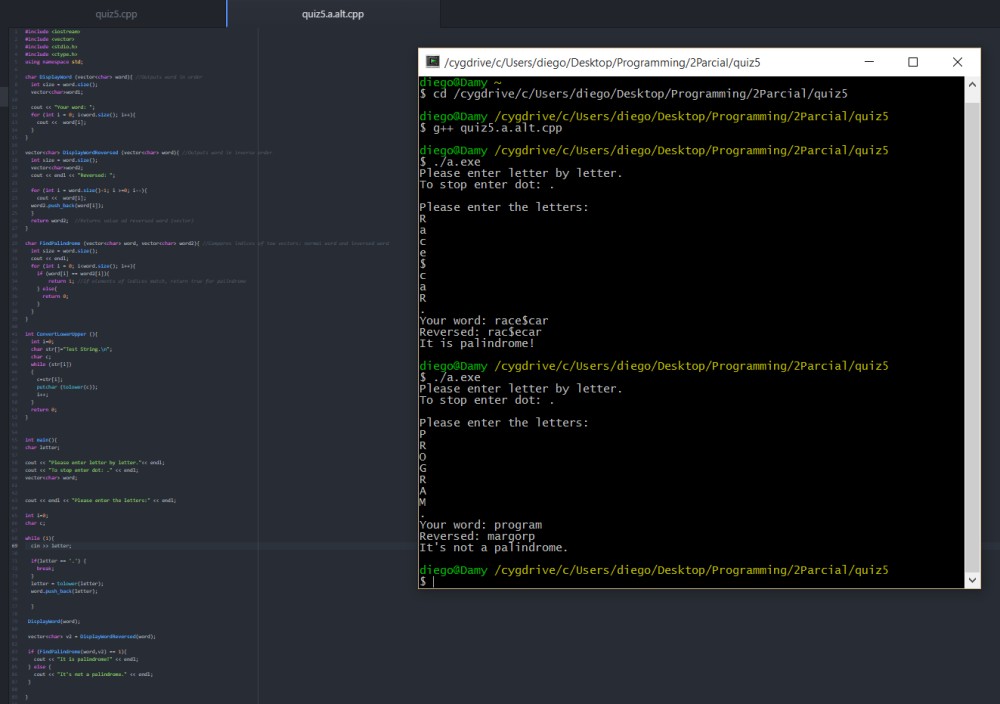
Source Code below:[GitHub Link: https://github.com/diegodamy/Quiz5%5D
#include <iostream>
#include <vector>
#include <stdio.h>
#include <ctype.h>
using namespace std;
char DisplayWord (vector<char> word){ //Outputs word in order
int size = word.size();
vector<char>word1;
cout << “Your word: “;
for (int i = 0; i<word.size(); i++){
cout << word[i];
}
}
vector<char> DisplayWordReversed (vector<char> word){ //Outputs word in inverse order
int size = word.size();
vector<char>word2;
cout << endl << “Reversed: “;
for (int i = word.size()-1; i >=0; i–){
cout << word[i];
word2.push_back(word[i]);
}
return word2; //Returns value od reversed word (vector)
}
char FindPalindrome (vector<char> word, vector<char> word2){ //Compares indices of tow vectors: normal word and inversed word
int size = word.size();
cout << endl;
for (int i = 0; i<word.size(); i++){
if (word[i] == word2[i]){
return 1; //If elements of indices match, return true for palindrome
} else{
return 0;
}
}
}
int ConvertLowerUpper (){
int i=0;
char str[]=”Test String.n”;
char c;
while (str[i])
{
c=str[i];
putchar (tolower(c));
i++;
}
return 0;
}
int main(){
char letter;
cout << “Please enter letter by
Continue reading “Palindroming all day long” →