Simplicity is the ultimate sophistication.DaVinci
Hello people. I made my third and the last one of the first partial. We have to create two programs: the first program, may calculate the distance between two points (x1,y1) & (x2,y2). In the second one, we have to create the Fibonacci sequence on C++ and ask to the user for the value that he/she wants. To make that, we use our previous knowledge about it, like conditionals, loops, functions, etc. so it wasn’t difficult.
The instructions were:
1. Write a function called distance(x1, y1, x2, y2) which receives four numbers which represent two points in the cartesian plane. The function should return the distance between the two points (x1,y1) and (x2,y2). Remember that the square of the hypotenuse of a right angle triangle is equal to the sum of the squares of the two other sides. Also, don’t forget to declare the variables with FLOAT to use decimal numbers.
**The new thing here, was the use of the math library, to can execute the square (SQRT in C++). The math library is using like this:
#include <cmath>
And now you can use the formula of the Pitagoras Theorem :
Distance= sqrt((x2-x1)^2+(y2-y1)^2)
Here my code with comments to make it understandable.
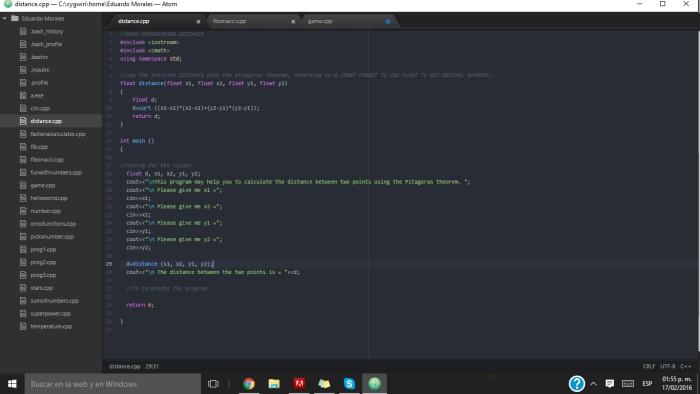
And the program running:
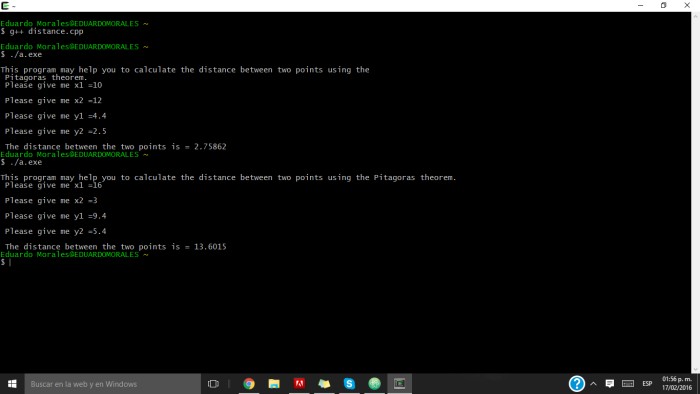
2. Write a function called fibonacci which receives a single parameter “n” (a non-negative integer) and returns the nth number in the fibonacci series which is: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89………… So, fibonacci(0) would return 0. fibonacci(5) would return 5 fibonacci(8) would return 21. Note that the first two fibonacci numbers are 0 and 1. All others are the sum of the previous two fibonacci numbers. int fibonacci(int n){ }
Here I got a headache with the Fibonacci Formula, but once
Continue reading “Quiz 3” →