Yes. I know, the “fun/funnier/funniest” pun, It’s done, get over it.
Today’s practice is about factorials. In math, these are expressed as “n!” and the expression equivalent to it is n! = (n)*(n-1)*(n-2)… and so on, until 1. for example 3! = (3)(2)(1) = 6, 4! = (4)(3)(2)(1) = 24, and 5! = (5)(4)(3)(2)(1) = 120.
So… As you might expect, our code needs to take an input an produce it’s factorial as an output. We can do this with a loop… or recursively, and yes, I’ll go over both.
Factorial using loops is kind of the easy way and… It works best for your computer, let’s just say you’ll save your computer a lot of resources. Plus, we kind of already did this with loops, let’s open up wsq07, remove one of the limits and rename the other one as the user’s input, replace the initial value of c with 1 instead of 0, and inside the loop replace the addition with multiplication. Like this :
————————————————————————–
Editor :
Terminal :
————————————————————————–
Great! it works. What pass does is literally nothing, we just don’t want i to multiply c when it equals 0, range(1, a+1) would work as well. What if we want to use a while loop instead of a for?
Well, we’d have to use c as a counter instead of a printable variable and use the input variable as the printable variable :
————————————————————————–
Editor :
Terminal :
————————————————————————–
c -= 1 is the same as c = c -1. We use c > 1 as conditional instead of c > 0, because when c = 1, then the loop’s output would be 0.
Our time is now. Actually… not our time, it’s more like… recursion time. Python supports recursion, this
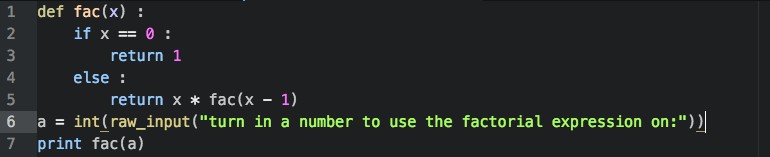

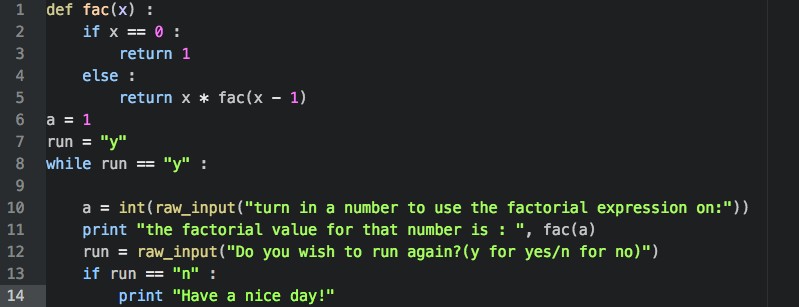



you can actually change a variable’s value in terms of it’s former value as in c = c -1, and this doesn’t only apply to variables, but also to functions. Functions can be defined in terms of itself, and conveniently one of the most used examples to explain recursion in python is the recursion function. Let’s remember what recursion does… the number multiplied by the number itself minus 1, multiplied by itself minus the number minus 2 until the expression the expression “n minus a number” equals 1, at that point the operation stops and the result is returned. And the way of representing this inside a function in python is this :
————————————————————————–
Editor :
Terminal :
————————————————————————–
The function is defined calling itself. it multiplies it’s parameter by the function itself called on the original parameter minus one. The condition that ends the recursion is x == 0. When we achieve this point, it is understood that x has been multiplied by each value of the function called over it until 1. It is important that we use return and not print inside the function, this is what makes recursion work.
Just for fun, let’s give our code some user friendliness with a loop and some strings.
————————————————————————–
Editor :
Terminal :
————————————————————————–
Great, now our code is user-friendly 😉
This post is for my #WSQ09 on the programming fundamentals course.
#WSQ09 – Fahnniest with numbahs by esenombredeusua is licensed under a Creative Commons Attribution-ShareAlike 4.0 International License.