Ok, we’re doing it really fine, but now, it’s time to combine while loops with conditionals.
(I needed help in this task, so my friend David García (he finished this course already last semester, here’s his wordpress blog link: David’s Blog) helped me to finish this task.
Also my friend Héctor Bueno helped me, here’s his blog: Héctor’s Blog.
Here’s what we have to do:
- Write a program that asks for a range of integers and then prints the sum of the numbers in that range (inclusive).
- You can use a formula to calculate this of course but what we want you to do here is practice using a loop to do repetitive work.
- For example, the sum from 6 to 10 would be 0 + 6 + 7 + 8 + 9 + 10.
Let’s begin:
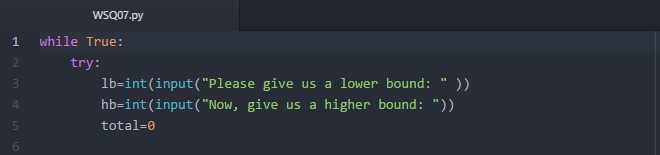
We have to open a while loop that will keep repeating until the program reaches the range we defined for the program.
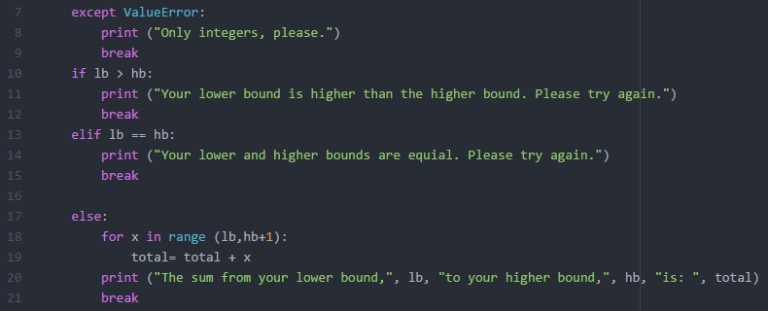
The “ValueError” works for people who tried to insert a variable instead of a integer, and also, breaks the loop.
The first condition (“if”) works for people who type a more bigger number in the lower bound than in the higher bound, making the range imposible to calculate. This condition also breaks up the loop too.
The second condition (“elif”) works for people who type the same number as lower and higher bound, making the range imposible to calculate too. This conditions (yes, again) breaks up the loop.
The third condition (“else”) (the good one) works for people who type things right and includes numbers from the lower bound number (lb) to higher bound number (hb) (+1 is for the higher bound number to be included in the range). The loop repeats until it reaches the higher bound number making
Continue reading “#WSQ07 – Sum of Numbers” →