So this has been the easiest task and blogpost so far! They say that if you work hard at the beggining it will help you at the end, and it sure is paying off.
I mentioned all that because this program is exactly the same I had to do on #WSQ03, but now using functions. The cool thing is, when I did #WSQ03 I used functions in order to practice! So basically you´ll see the same program here. BTW, the image above is how this program looks when you run it.
Functions are really cool tools that you can use in order to put your program together in a more organized way. In order to use them, you have to build them first. You usually build them before the code inside main that “calls” the funtion, and before the main, which is a function as well actually.
To call the function, you just have to write the function name with a parenteses. Inside the parenteses you have to write the parameters, which are the values or information that the function is going to work with.
Here you can have a look at my code:
#include <iostream>
using namespace std;
int x, y;
int multiplication(int z, int p){
int operation = z * p;
return operation;
}
int divition (int z, int p){
int operation = z / p;
return operation;
}
int sum (int z, int p){
int operation = z + p;
return operation;
}
int subs (int z, int p){
int operation = z – p;
return operation;
}
int remainder (int z, int p){
int operation = z % p;
return operation;
}
int main(){
cout << “This program will do some basic operations with two numbers. Please provide the first number” << endl;
cin >> x;
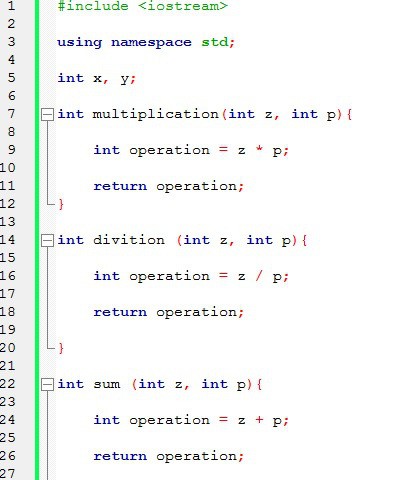
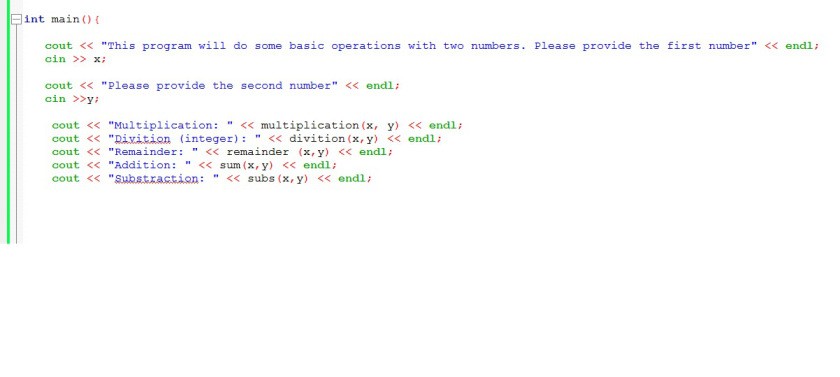

