--Originally published at hermesespinola
This thing was really weird, I mean, it’s useless but it was interesting.
So a lychrel number is a special number, if the repetitive addition of a number with the number formed with inverse of its digits form a palindrome then it’s not a lychrel number.
So, in example: 196 + 691 = 887, 887 + 788 = 1575, … and so forth.
So here I first programmed the YoSoy186 class, before programming LychrelSequence and LychrelNumber, because I wanted to define how the class would work instead of programming it in the first place.
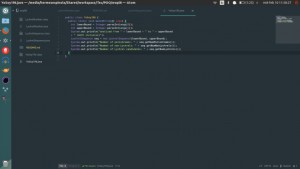
So because I needed to flip the digits of the numbers, I converted the number to a String. And because in this lynda Java course they said I should use the StringBuilder class to work with string, because it’s expensive to do the string operations with the normal String operators.
And then, in the Lychrel number class, I wrote a lot some fields, getters and setter then I wrote the above algorithm to evaluate if the number is a candidate to be a lychrel number:
public class LychrelNumber {
private int value;
private boolean isPalindromeNumber;
private boolean isLychrel;
public LychrelNumber() {
}
public LychrelNumber(int number) {
setValue(number);
}
public boolean isLychrelNumber() {
return this.isLychrel;
}
public boolean isPalindrome() {
return this.isPalindromeNumber;
}
public int getValue() {
return this.value;
}
private long[] evaluateHelper(long val) {
StringBuilder reverseStr = (new StringBuilder(Long.
toString(val))).reverse();
long reverse = Long.parseLong(reverseStr.toString());
long add = val + reverse;
return new long[] {reverse, add};
}
private void evaluate(long value) {
long[] r = this.evaluateHelper(value);
String addStr = Long.toString(r[1]);
this.isPalindromeNumber = (value == r[0]);
if (!this.isPalindrome()) {
for (int i = 0; i < 30; i++) {
r = evaluateHelper(r[1]);
addStr = Long.toString(r[1]);
if (addStr.equals(new StringBuilder(addStr).reverse().toString())) {
this.isLychrel = false;
break;
}
this.isLychrel = true;
}
}
}
public void setValue(int number) {
this.value = number;
this.evaluate((long)this.value);
}
}
In the code I wrote a private method, which is only called when the setter of the value is called, and another method to reverse and add these numbers. I used a long number because the repetitive addition of the numbers generated huge numbers and the program would run out of memory.
So first, before checking if the number is a lychrel number, I check if it is a palindrome, and then, if it is not, run the code to check if it is a lychrel number (because if it is a palindrome then it’s not a lychrel number). If at some point the addition of the number and the inverse of its digits is a palindrome, the code breaks and sets the isLychrel number variable to true.
For the LychrelNumber class, I used the ArrayList class to be able to append elements to the list, something you can’t do to a normal array easily. I used three lists, for storing the lychrel, non-lychrel, and palindrome sequences. The link says that since the List class is an Interface, you need to declare a specific implementation of this Interface.
All this class does is iterate from the lower bound to the upper bound, and instantiating the LychrelSequence class with that value, and if the number is a palindrome, it’s added to the palindromes list and the non-lychrel list, else, if the number is a lychrel number it is added to the lychrel list, but not all non-lychrel numbers are palindromes).
import java.util.List;
import java.util.ArrayList;
public class LychrelSequence {
private int lower;
private int upper;
private List<Integer> lyqSeq;
private List<Integer> nonLyqSeq;
private List<Integer> palindromeSeq;
public LychrelSequence(int lowerBound, int upperBound) {
this.lower = lowerBound;
this.upper = upperBound;
this.lyqSeq = new ArrayList<>();
this.nonLyqSeq = new ArrayList<>();
this.palindromeSeq = new ArrayList<>();
LychrelNumber ln = new LychrelNumber();
for (int i = this.lower; i <= this.upper; i++) {
ln.setValue(i);
if (ln.isPalindrome()) {
palindromeSeq.add(ln.getValue());
nonLyqSeq.add(ln.getValue());
} else if (ln.isLychrelNumber()) {
lyqSeq.add(ln.getValue());
System.out.println(“Found a Lychrel number candidate: ” + ln.getValue());
} else {
nonLyqSeq.add(ln.getValue());
}
}
}
public int getLowerBound() {
return this.lower;
}
public int getUpperBound() {
return this.upper;
}
public List<Integer> getPalindromeSequence() {
return this.palindromeSeq;
}
public List<Integer> getLychrelSequence() {
return this.lyqSeq;
}
public List<Integer> getNonLychrelSequece() {
return this.nonLyqSeq;
}
public int getNumPalindromes() {
return this.palindromeSeq.size();
}
public int getNumLychrels() {
return this.lyqSeq.size();
}
public int getNumNonLychrels() {
return this.nonLyqSeq.size();
}
}
Something weird I noticed is that you have to use the Integer class when declaring the list, instead of the primitive type int.
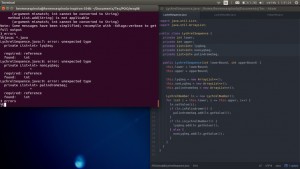
And the here’s the Github repository of this homework.