--Originally published at Blog
In this post I’ll show the most basic usage of the Unity3d game engine, using the C# programming language, its syntax is very similar to Java.
I’ll be using public domain assets that I downloaded here.
And instead of using the default cube of Unity, I’ll use this one, because it’s made out of Quads (like a plane, you can only see it from one side), and it’s easier to add the textures to it (I don’t know how to use blender and stuff).
Ok, so now open Unity (which you can download from it’s page), and create a new 3D project, by default, it only has a camera.
So, the Editor has windows, and you can move them around, you can add new ones in the Window top menu. The ones I’ll need are Game (here you see what the cameras are projecting), the Scene (where you edit the game), the Inspector (where you see the selected object’s properties), the Hierarchy (where you see and select objects in you scene), the Project (like a file manager), and the Console (here you see errors and warnings).
In the Project and Hierarchy windows, there is a drop-down button that reads: “create”. when you click it, you will see a lot of pre-build thing that you can use in the editor, and whatever you crete, it will appear inside the window where you clicked “create”. When I say “create something in bla”, I mean that you should click “the” bla button in this drop-down, inside the “something” window.
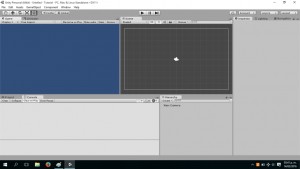
Extract the and drag and drop the PNG folder into the Project window. Make sure you organize your files. Create these folders (right-click > create > folder):
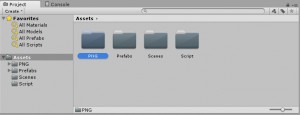
In the Hierarchy window click on Create > Light > Directional light, this light is behaves like a sun, it doesn’t matter where you put it, only it’s rotation.
Put the Cube.prefab file in the Prefabs folder (does it makes sense?) and then drang and drop that weird thing to the Scene window, you’ll that the cube appears magically in the Scene, and also, the cube is added to the Hierarchy window.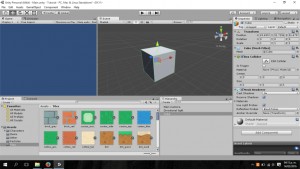
Open the Tiles folder in the PNG folder, now, if you drag and drop the images in the cube that’s in the scene, you can assign that texture to the side of the cube where you drop the image (and that’s the reason behind the special cube prefab). You can see that, in the Hierarchy, there’s an arrow next to the cube, if you click that arrow, you will see it’s children.
GameObjects can have children, which are also GameObjects. Children have a relative position to it’s parent, and they can be easily referenced from a script. For example, you can have the “player” object, and a “gun” object. You want that gun to be a child of you player, so it’s always, in it’s hand.
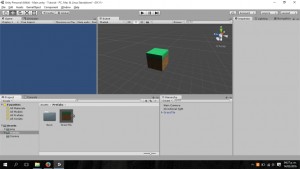
Now add a RigidBody to the tile, this will allow the tile interact with other objects, and also, deselect the “use gravity” button.
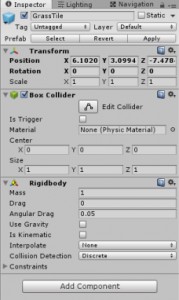
Once you’ve done this, go to the Prefabs folder and (in the Project window) click on Create > Prefab. Now, drag and drop the Cube (in the Hirarchy window) to this new Prefab in the Project window. As you can see, the cube is “inside” the prefab. you can now delete the cube from the scene.
A prefab is the way Unity defines custom Game Objects, so that you can instantiate them, without the need to create them again, so it’s very similar to creating a class when you are programming (but of course, it’s not the same).
create an empty game object in the Hierarchy, and name it, I don’t know, map, or terrain, or whatever, then go to the Script folder and create a new C# script, we’ll use this script to automatically generate the terrain, based on the tile I’ve just created, when the game is loaded. drag and drop this new script to the empty game object in the Hierarchy.
Every GameObject you create has different fields, if some field is set to public, it will appear in the Inspector window, you can change the values of these properties inside the Inspector.
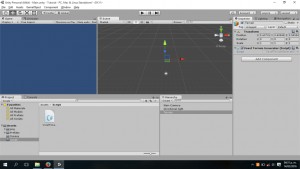
Everything in Unity is a GameObject, which is a special class of Unity, and every GameObject has at least a Transform. a Transform determines the position and rotation of that object in the scene.
If you double click the script you’ve just created, the code editor should be opened (Visual Studio or Monodevelop).

The C# syntax is very similar to Java, but to implement and interface you write <ClassName> : <InterfaceName>.
Monobehaviour is a special interface of Unity, if you delete that, you will not be able to attach the script to a game object.
In Unity Scripts, there are special method names, the Start method is called when the GameObject is instantiated and the Update is called once every frame. You can search for more special names in the Unity documentation.
add these fields, you want them to be public so you can change them from the Unity editor. the grassTile of type Transform will hold the prefab I created earlier. the tileSize is set to 1 by default, but you can change it from the editor. You need to drag and drop the tile prefab we created to the Grass Tile field that you can see in the Inspector when the Terrain object is selected.
There are Vector2 and Vector3 classes, and they are what you would expect.
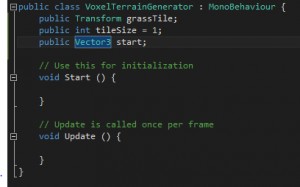
Now add a Vector2 called terrainSize and these for loops:
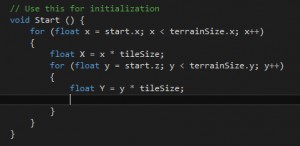
I’ll create a plain terrain for now, the terrainSize represents the amount of tiles with size = tileSize. These for loops will “walk” through a “grid”, in each position a new tile will be instantiated:
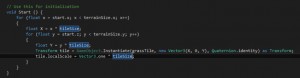
This is a static method in the GameObject class that creates the specified object in the scene (and you can instantiate almost anything, because everything is a GameObject). It returns the instantiated object, and we can tell C# to convert it to a Transform (because aTransform is a Game Object, and you know, “polymorphism” stuff).
You can change the values of the public fields that you declarated in the script:
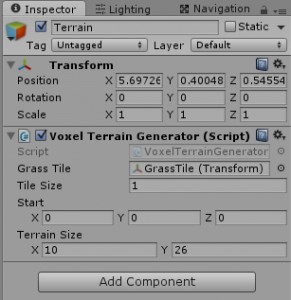
You should see something like this in the Scene window.
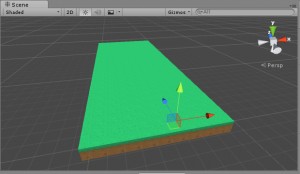