--Originally published at angelmendozas
Python has five basic types:
#Numbers
you can put them as:
a = 1 b = 3 or var_1=4 var_2=3 or delete them like (“del var”) or (“del var1[,var2[,var3]]] etc.
Also python supports four numerical types:
int (integers), long (long integers, can also be octal or hexadecimal), float (floating point real values) and complex (complex numbers).
#String
A string’s official definition is: “Strings in Python are identified as a contiguous set of characters represented in the quotation marks. Python allows for either pairs of single or double quotes. Subsets of strings can be taken using the slice operator ([ ] and [:] ) with indexes starting at 0 in the beginning of the string and working their way from -1 at the end.”
I made several strings, sentences to link one to another and printed them to have fun and made up sentences combined with the strings.
The results were these (Elizabeth is a random name, don’t worry):
#List
“Lists are the most versatile of Python’s compound data types. A list contains items separated by commas and enclosed within square brackets ([]). To some extent, lists are similar to arrays in C. One difference between them is that all the items belonging to a list can be of different data type.” -tutorialspoint.com
I made a basic list with words
this is the result, it solved the 17×10^-4 and the other values were just put like they were in the list
#Tuple
Tuples are the same as a list its just that the variables go inside of () instead of []
#Dictionary
According to tutorials point: “Python’s dictionaries are kind of hash table type. They work like associative arrays or hashes found in Perl and consist of key-value pairs. A dictionary key can be almost any Python type,
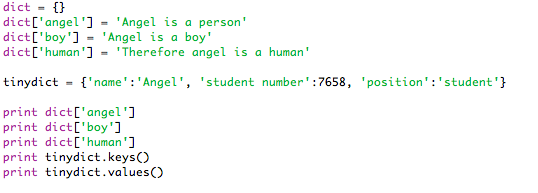
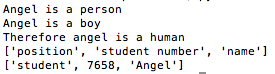
