--Originally published at Programming
If-Statement
It’s time for us to talk about the conditional statements. First we are going to talk about the if statement. the if statement is a way to make your computer program make a very simple decision. An if-statement is followed by an indented block of code that is run if and only if the expression is true. Say we want to make a program that asks for a person’s age and if his/her age is equal or greater than 18 we print a message to the screen.
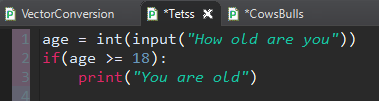
Expressions can be tested in several different ways. Here are some of the most common ones:
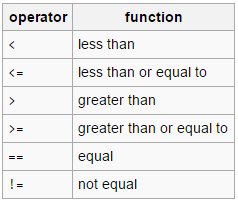
*Note: When comparing two strings or numbers you can use the keyword is to check if they are the same.
Else-Statement
If the expression within the if statement is false, then the program will look for an else statement, which is the statement that will be run whenever the if statement is false. Working with the same example, we want to tell the people whose age is not greater or equal than 18 that they are too young.
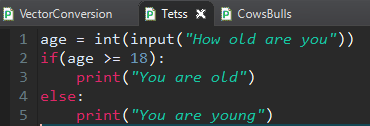
Elif-Statements
The elif statement allows you to check multiple expressions and as soon as the condition evaluates to true, the program will execute a block of code. The elif statement is optional and can be written several times after an if statement. Let’s make an example where the user enters his Grade and the computer prints it using the American Grading System.
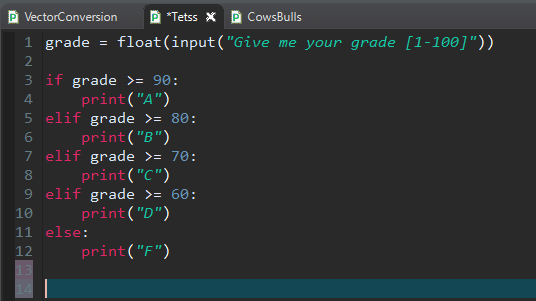
Nested Conditional Statements
Sometimes in a program, you are going to need to check another conditional after the previous condition is resolved to True. When that happens, you are going to need to use nested if statements. Let’s take a look at an example. We are going to make a program that prints to the console the bigger
Continue reading "Conditional statements" →