--Originally published at PYTHON 3
What is a module?
A module is an object that lets you organize your Python code.
A file consisting of python code, this can define functions classes and also variables, and it also can include runnable code.
Any python file “something.py” can be use as a module by executing the next code in another or brand new python file.
Import module1[, module2[, …..Module(n)]
In other words, a module lets us import directions/ functions / defined functions from another python file, and instead of writing the same commands on our new Python file, we just need to put our directions for example:
In the last blog I learned how to create and call functions, so in my file “creatingandcallingfunctions.py” I already have myinstruction /function“repeat()”
which prints a lot of lines with numbers, so in my new file “import.py” I just need to import.
Import creatingandcallingfuncitons
##It is very important that the files you are going to import are in the same directory as your new file, because that’s the way it works.
But the problem in this is that you may also import other modules which have a different function but with the same name, so you have to define from what file you are going to import that function
import creatingandcallingfuntions
creatingandcallingfunctions.repeat()
creatingandcallingfuncitons.repeat()
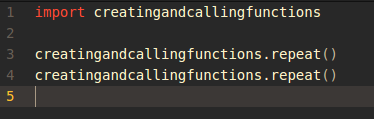
In my file “creatingandcallingfuntions.py” I already had instructions, which were printing 2 sets of numbers, and in this new file i[m importing this instructions and also giving the directions to import another 2 sets of numbers, so in total there should 4 sets of numbers.

Here is the link were I learned about modules:
http://www.tutorialspoint.com/python/python_modules.htm