--Originally published at TEC GDL 2016
Today I will complete the mastery topic of the basic types there are in Python. Please make sure to comment of write me via Twitter (@tecjames) if you have any questions about this.
- Lists
Lists in Python are awesome because, compared to other software languages, a list can contain items of different types and Python keeps track of the datatype internally.
Lists within Python are assigned several items separated by commas (,) and the whole list is enclosed by square brackets ([]). You can then easily print either the whole list or different parts of the list (denoting print(my_list) or print(my_list[2] ). See how this works below:
When choosing the value 0 to print, Python will always use the first item in your list, as lists are zero-based. When choosing to print out e.g. [-3], Python will count “backwards” and (making use of my program) display “example”.
The values can be accessed using a so-called slice operator ([]) and ([:]). Thus, you can choose to only print specific parts or “slices” of your created list. When writing e.g. print(my_list[1:4]), the output will include a new list, starting with the first item called (1) up to, but not including the second value (4).Similarly, other possible options can be handled easily. Have a look below to see these rather straightforward prints.
There are many more things you can do within the list type. If you would like a better idea of how lists can be used apart from what I have covered, please visit this LINK covering also how to add or remove items or how to search for values within a list.
- Tuples
A tuple is similar to a list. The main difference to normal lists as covered before is, that tuples cannot be

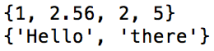

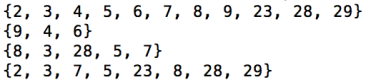
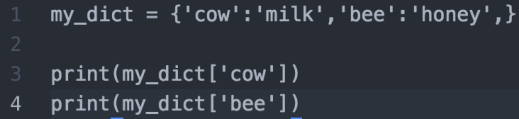



Why do we use tuples then?
Well, tuples are faster and safer to use within your code and they can be used as dictionary keys.
By using the list() or tuple() functions, lists can be easily converted into tuples and vice-versa.
- Sets
A set can be defined as an ‘unordered bag of unique elements’. A set can contain values of any immutable data types (Numeric types (int, float, complex), String, Tuple, frozen set & bytes). But, compared to lists, sets are unordered and cannot contain duplicates of elements.)
[For more information on mutable and immutable data types please visit THIS site]
How to create a set is quite straightforward. This is done as follows:
To add values to a set, one can use the add or update method. In the program above this would mean you could either write my_set.add(3) (for single elements added) or you could choose to write my_set.update([3,4,5]) for multiple values.You can delete items off a set through using either my_set.discard(3) or my_set.remove(3). You can ask Python if a certain value is within a list by using the “in” operator (e.g. 1 in my_set –> True).
- Sets can perform different operations like intersections, union, difference and symmetric difference. With union, you are able to check which items are in either set and with intersection you can check which items are in both sets. Additionally, difference allows you to display all elements within a_set but NOT in b_set and symmetric_difference, returns a new set containing all elements that are in exactly one set.
- Dictionaries
A dictionary is an unordered set of key-value pairs, meaning that we pair e.g. the word ‘cow’ (key) with ‘milk’ (value). Once the dictionary is set up, you can look up values by their keys (doesn’t work the other way around). A dictionary is set up as follows:
If you wish to change a key-value pair, make use of this:
Dictionary values can be any type (integers, booleans or other dictionaries). For keys of dictionaries, you can use less, namely integers, strings and few other types.
______________________________________________________________
By covering lists, tuples, sets and dictionaries, together with my last blog post, I believe to have written about all main types that are used within Python.
PLEASE LET ME KNOW if this list is incomplete and I will gladly add another blog post regarding another type.
Let me know if you have any questions!! (@tecjames)
Best,
James
Sources:
http://www.diveintopython3.net/native-datatypes.html
Python Objects: Mutable vs. Immutable
https://www.dotnetperls.com/set-python
https://www.tutorialspoint.com//python/python_variable_types.htm
