--Originally published at Programming
Introduction
This is going to be the last blog post for this semester’s introduction to programming using Python. Today we are going to talk about files, how to create a file, how to read a file and how to write on a file.
Create a file
To create a file, we need a file object. In order to do that, you need to create a variable, name it however you want, and set it equal to the built-in function open(), which takes two parameters. The first parameter is the name of the file, if the file already exists, Python will use it and if it doesn’t exist, it’ll be created. The second parameter is going to take a character which might be ‘w’ or ‘r’. The ‘w’ character means you are going to be writing to the file you’ve created. The ‘r’ characters means you are going to be reading the contents of the file. At this point you have created a file object.
Write on File
In order to write on the file we’ve just created, we have to use the file method write(). Basically this method is going to allow us to type on the file. This method takes one parameter, which is the text you are going to write on the file. After you hit the run button, you will find a .txt file in the folder where your .py file is. After you are done writing, you must close your file object by using the close() method so you don’t waste any extra memory.
Read From a File
In order to read from a file, another file object is going to be required. First you name your variable, then you set it equal to the function open(). The same function that we used in order to create our writable

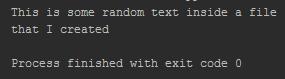

Relevant File Methods
- close() Closes the file
- read() Reads the content of your file
- readline() Reads just one line of your file
- truncate(size) Empties file
- write(‘something’) Writes ‘something’ to your file
