--Originally published at My B10g
Most of the times that you create a program you expect it to interact with the user, and by doing so they may be doing things that they aren’t supposed to, like writing word where they should write numbers or use special characters in strings, in this cases the computer will drop an error and will stop the program but how to prevent it from stoping and just let the user know he is doing something wrong? that is exactly what this topic is about.
As in many things in programing how you do it depends on the programer and there are plenty of ways to do so, so I will be explaining just the basic concept and to do so I will use an example.
def get_int(prompt):
try:
value = int(input(prompt))
except ValueError:
print("Sorry, I didn't understand that.")
return get_int(prompt)
if value < 0:
print("Sorry, your response must not be negative.")
return get_int(prompt)
else:
return value
to get started we need to know that we will need to create a loop (in this case the loop is created by calling the function again once it finds a mistake) because you want it to no matter how many times the user make a mistake the program should not stop until everything is correct, next we will need to check if the input is the correct type, to do so we will transform the input to an integer and in case it isn’t a number it would drop a mistake and for that we will use an except which will execute what should it do in case the program find a mistake of this type, in this
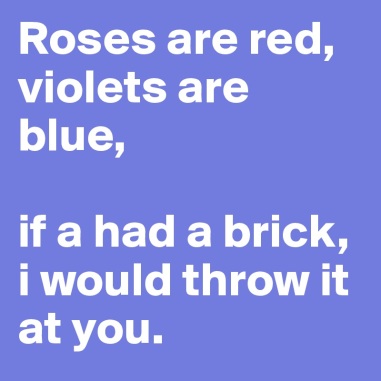
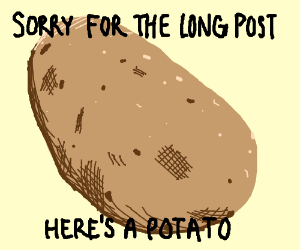

